- Published on
Singleton design pattern
- Authors
- Name
- Soulaimane Yahya
- @soulaimaneyh

In this article, we’ll take a deep dive into Singleton design pattern, exploring its key features and providing practical expls of how to use it.
Table Of Contents
- Singleton design pattern
- Global Access Point
- How to implement Singleton
- Creating Singleton class
- Conclusion
Singleton design pattern
The Singleton is a creational design pattern ensures that a class has only one instance and provides a global point of access to that instance. It's useful when you need a single, shared resource across the application, such as a configuration manager or a database connection.
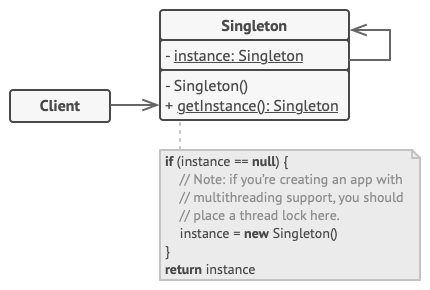
Global Access Point
A Singleton provides a global access point
to the instance. The class typically has a static method (often getInstance()) that returns the unique instance, allowing different parts of the application to access the same object.
How to implement Singleton
Controlling instance creation.
- Private Constructor (__construct): Prevents direct instantiation of the class.
- Private Clone (__clone): Prevents cloning of the class instance.
- static: Uses late static binding, allowing subclasses to have their own instance when
getInstance()
is called on them. - self: Refers to the class where the method is defined, so it does not support subclassing in the same way.
Two options of implementing a singleton
Early initialization - Eager Singleton
Create singleton as soon as class is loaded
Lazy initialization - Lazy Singleton
Singleton is created when it is first required
Creating Singleton class
class MultiChatContainer
{
private static $instance = null;
// Private constructor to prevent direct instantiation
private function __construct()
{
}
// Private __clone to prevent cloning
private function __clone()
{
}
/**
* Get the globally available instance of the container.
*
* @return static
*/
public static function getInstance(): static
{
if (is_null(static::$instance)) {
static::$instance = new static;
}
return static::$instance;
}
}
$app = MultiChatContainer::getInstance();
Giving access to a Singleton
instance throughout the application, using public static method getInstance()
.
Additional Resources
Conclusion
As software engineers, we use design patterns daily, this improves readability and lower maintenance cost. We use the singleton pattern to make sure we have the same instance when we resolve it.